Build LLM-driven applications with Next.js, Vercel and Graphlit
Kirk Marple
July 30, 2024
We have built three new sample applications, which show the capabilities of Graphlit integrated with Next.js, and deployable on Vercel.
Each of this applications uses the Graphlit Node.js SDK (NPM) for integration with the Graphlit Platform API.
Also, each are deployable to Vercel, via the Deploy button on the README page of each Github repository.

Chat Application [Github]:
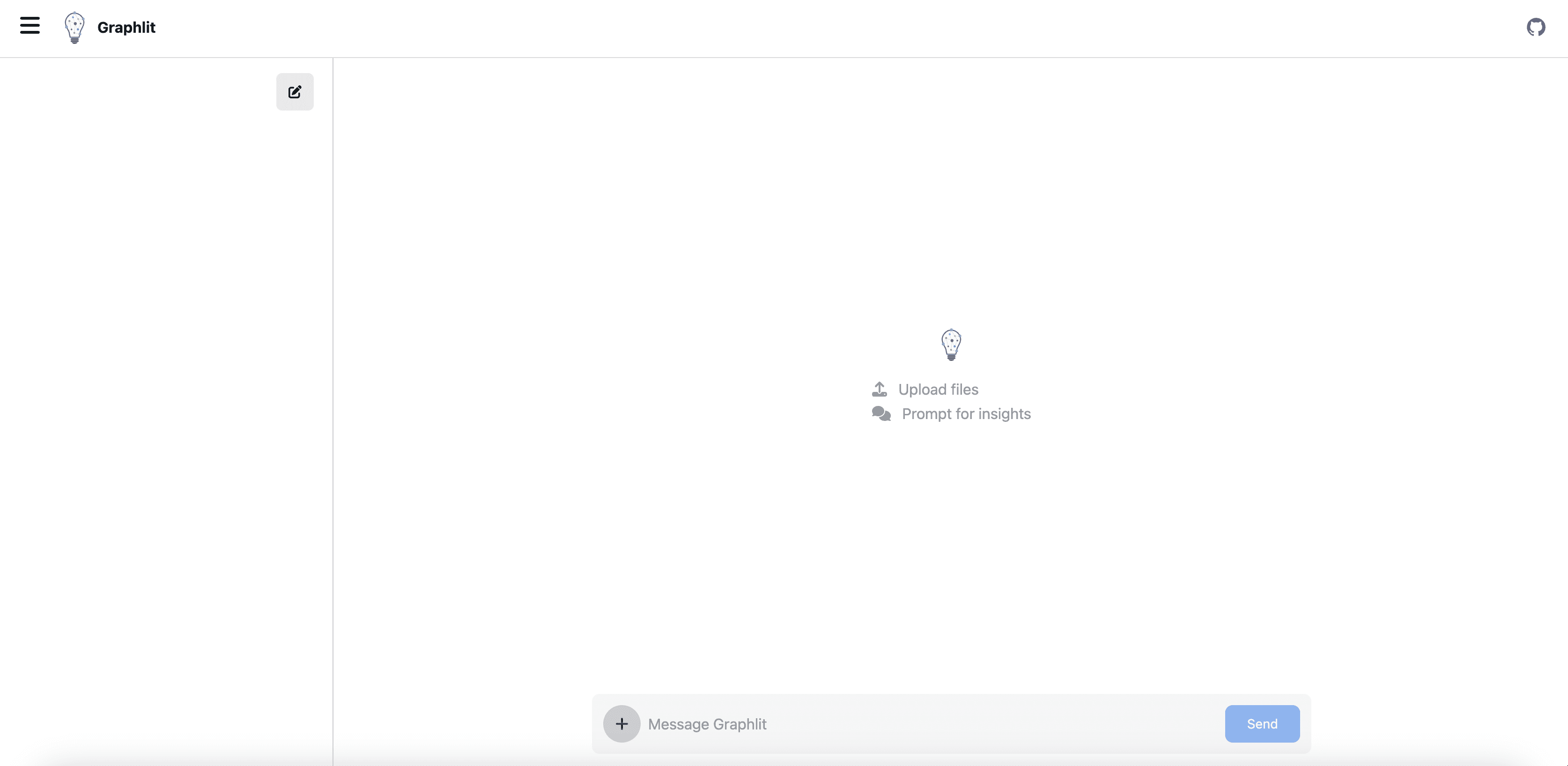
Similar to ChatGPT, Graphlit supports Retrieval Augmented Generation (RAG) conversations over ingested content.
In this application, you can upload one or more files to your Graphlit project, and then prompt a chat conversation to ask questions or summarize the file contents.
Files will be read from the local filesystem, and Base64-encoded before being sent to the Graphlit API. Ingestion runs synchrously given the isSynchronous
parameter is set to true
, and the API route will wait until the file has completed the ingestion workflow.
Then, when the user enters a prompt, it will be sent to the default LLM (OpenAI GPT-4o, at the time of publishing) for completion.
Previous conversations can be queried, and as a conversation is selected, the application loads the previous messages.
Web Extraction Application [Github]:

Graphlit can be used to scrape webpages or crawl websites and extract text, even without using the text for a RAG conversation.
Scrape
This sample application demonstrates how to scrape a webpage by URL, and then display the extracted Markdown text and structured JSON output.
Crawl
This sample application also demonstrates how to crawl a website by URL, walking the pages via sitemap. The application then displays the extracted Markdown text and structured JSON output of all crawled pages.
File Extraction Application [Github]:

In addition to extracting text from webpages, Graphlit supports extracting text from documents, such as PDFs and Word documents.
Similar to the Chat sample application, the File Extraction application demonstrates how to upload a local file. Once ingested, the application displays the extracted Markdown text and structured JSON output.
Summary
Please email any questions on this article or the Graphlit Platform to questions@graphlit.com.
For more information, you can read our Graphlit Documentation, visit our marketing site, or join our Discord community.